Javascript Block
The Javascript Block is the catch-all Block. It allows you to perform any task that is not covered by a Utility or Custom Block.
In place or full screen editing
There are two Javascript editing modes. You can edit in place in the Flow.
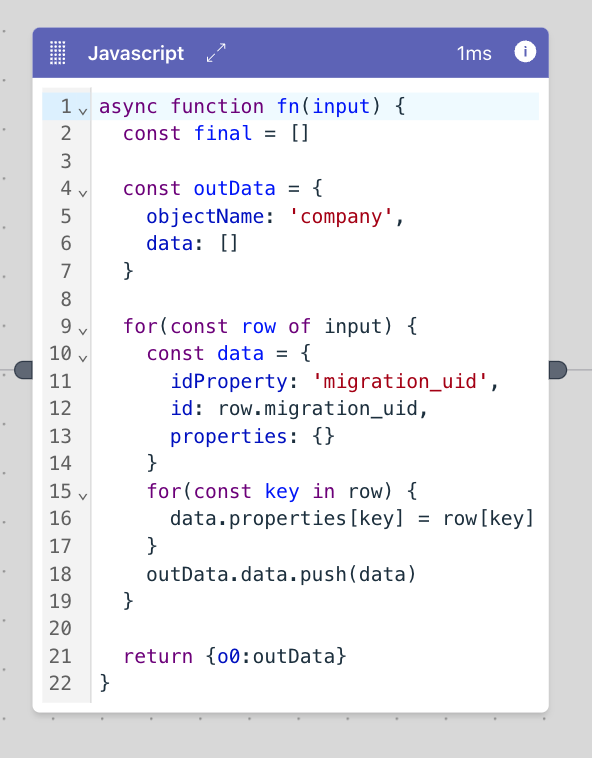
When your code gets too large for in-place editing, then press the expand icon in the Block header.
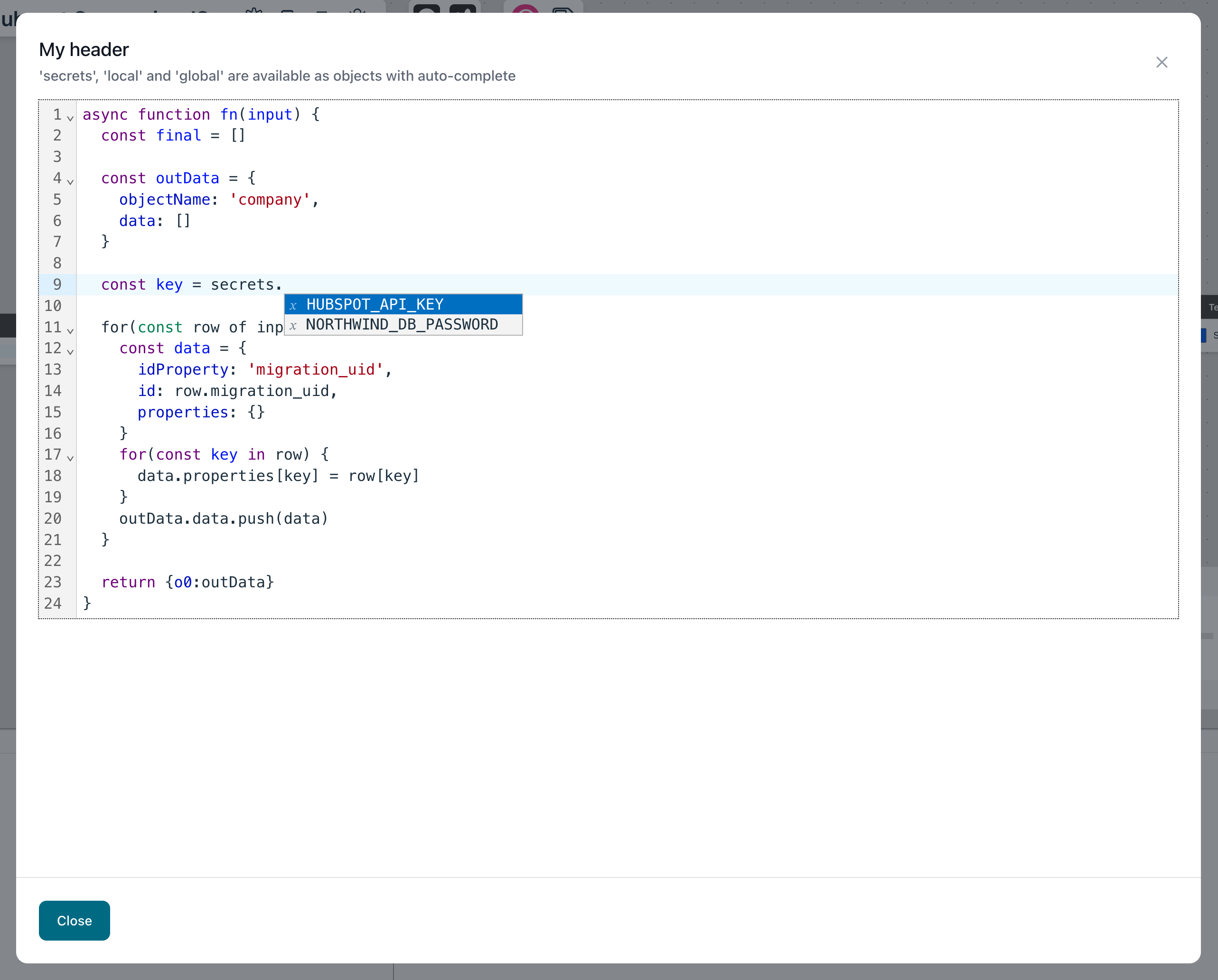
Inputs and Outputs
Inputs
Data is read from the incoming edge or edges. The Block will automatically add as many output edge connectors as there are input arguments. If you provide no arguments, then the edge data will be ignored.
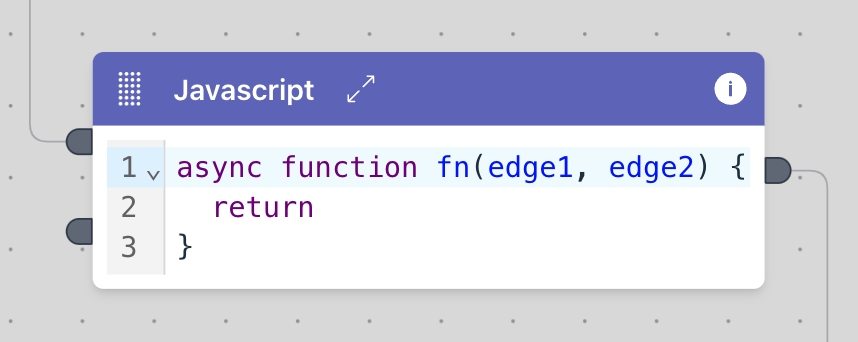
In the above screenshot, you can see two arguments and two corresponding input edge connectors.
Outputs
The return structure is examined to determine how many output edge connectors should be available.
If you have a simple return
statement, then an empty object is placed on a single output edge.
You can output a primitive as follows.
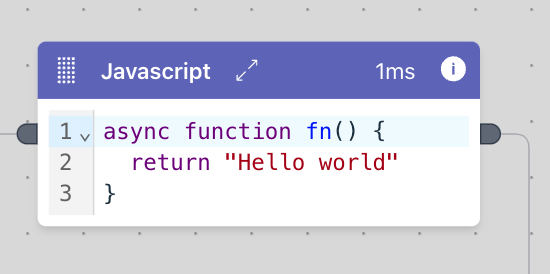
Or data on multiple edges.
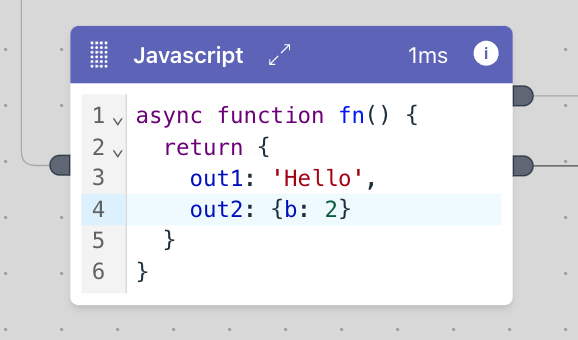
Branching to edges
You can handle any branching logic using the branchTo(edgeIndexZeroBased, data)
method.
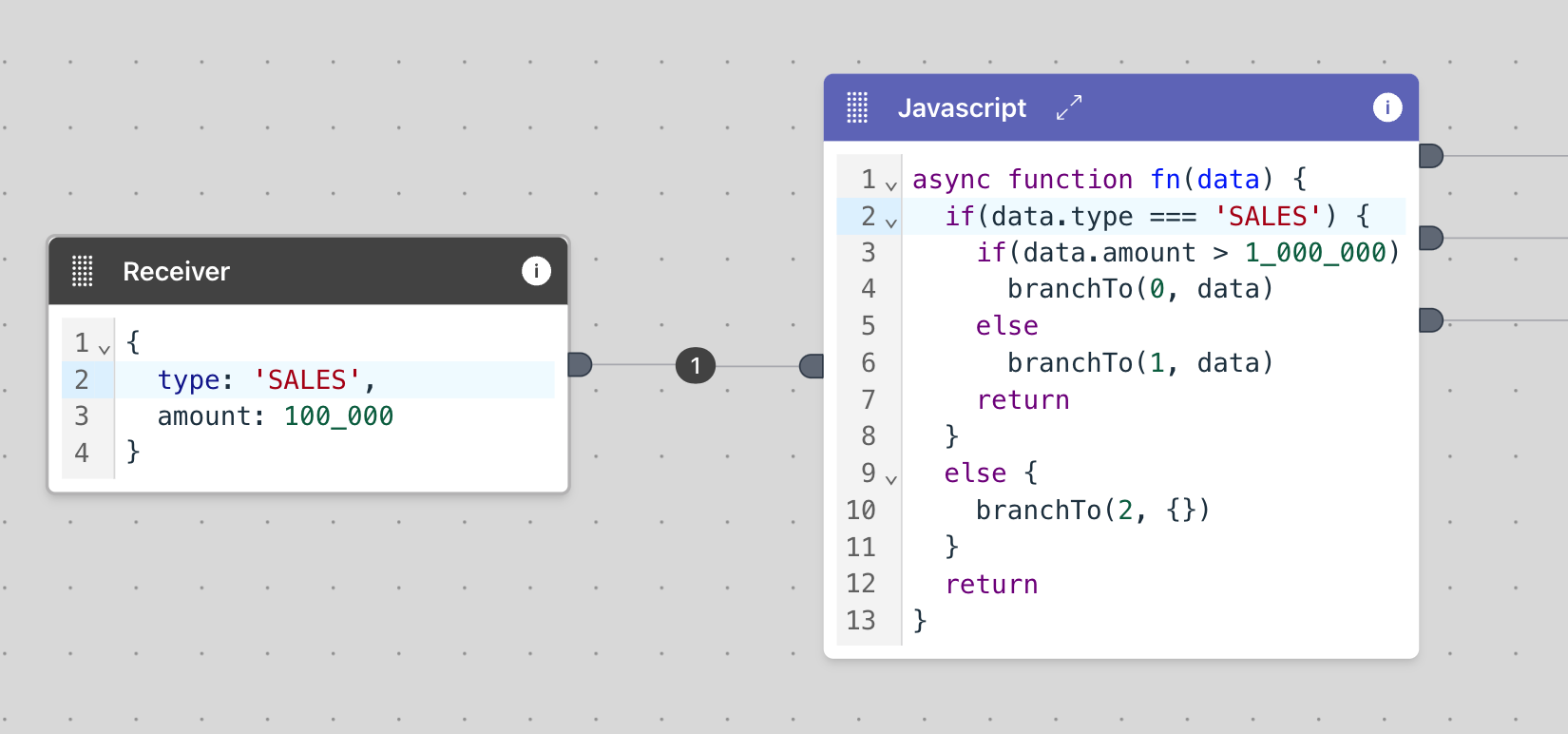
The output connectors will automatically be validated and created as you enter the branchTo()
commands.
You should not return any data using return {edge1: someObj}
when usiung branchTo()
.
Accessing Ziggy objects, values and methods
You can access various objects and values from the code editor. Basic auto-completion will help you find the object or value as well as available options for each one.
Console output
You can output information to the editor's console pane (bottom left).
Writing to system logs
You can also write to the Ziggy system logs. These are hourly rotated and are located in the /logs
folder.
Errors are logged as follows
All other log level calls .log()
, .warn()
, .debug()
, .verbose()
are as follows.
Connections
This will automatically select the development/production secret as determined by the prod/dev mode.
Secrets
Secrets can be accessed as follows.
This will automatically select the development/production secret as determined by the prod/dev mode.
Data Store
Refer to Data Store methods for available methods.
Memory Store
Refer to Memory Store methods for available methods.
Execution IDs
You can access the following execution IDs.
externalExecutionId
is an optional value that can be passed into the Flow when launched externally. This allows the calling system to provide a value associated with the execution for you to work with.executionCounter
is a simple sequential counter that resets to 0 when the Ziggy server restarts. It's main purpose is debugging and is not broadly useful.executionId
is a GUID for the individual execution. Again, the primary purpose is debugging.
Snooze
You can use the snooze()
method to pause execution for a specified number of milliseconds.
Custom client objects
You will have access to certain client objects. Which ones depends on your specific Ziggy configuration.
Assuming you have Postgres, SFTP and Hubspot clients available, you can access these using.
... where configObj
is specific to each one.
Batching
You can perform batching operations with the Javascript Block. Please refer to Batching for general information on Batching.
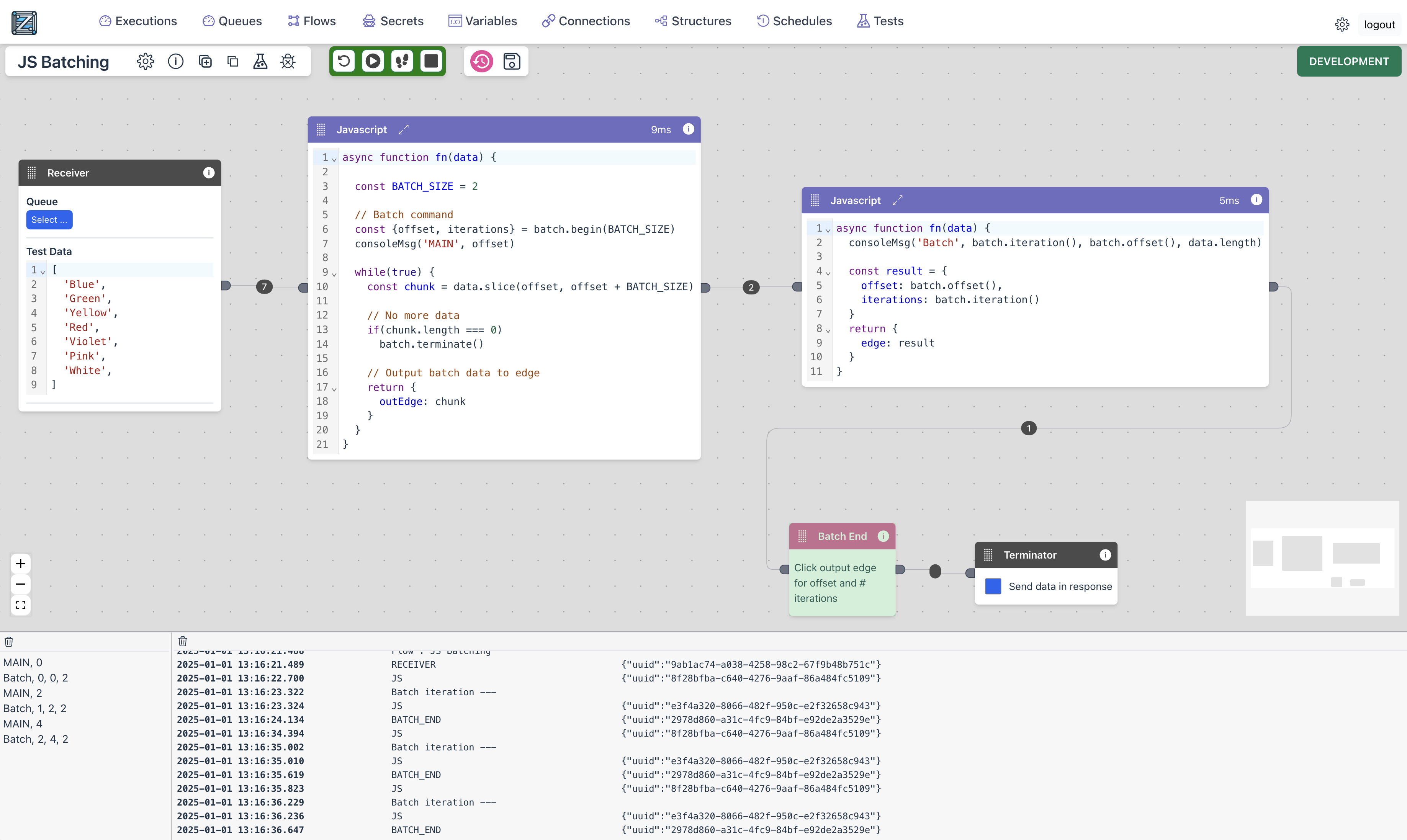
Available methods
You should use the batch
object, which has the the following methods.
batch.begin(batchSize)
- informs Ziggy that this is the starting point for batch operations and the size of each batch. This returns{offset: x, iteration: y}
wherex
is the number of records processed by the batch loops so far andy
is the batch iteration.batch.terminate()
- once there is no data left to process, call this to continue execution after the Batch End Block (or Terminator if there is no Batch End Block.)batch.iteration()
- returns the batch iteration counter.batch.offset()
- returns the current record # offset from the first batch, in other wordsbatchSize * batchIterations
.
Alerts
You can generate a custom alert. This adds an item to the Log and will also send an email alert.
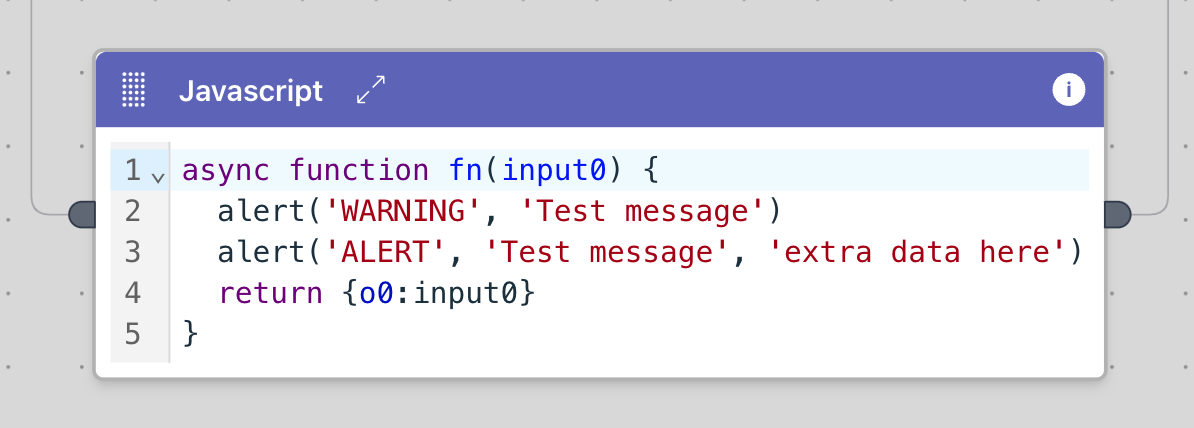
The first parameter (required) should be one of the following.
WARNING - this will not create an alert notification but it will show up in the Log.
ALERT - generates a log entry and sends an alert notification.
IMMEDIATE - sends an alert notifcation immediately rather than waiting for the digest notification to be sent.
The second parameter (required) is notification message.
The third, optional, parameter is any additional data you may want to add to the log. It will not appear in the notification.
Security
Script code does not have access to the following global objects.
However, the script code is considered trusted. This means that although there is good protection from harmful script code, it should not be considered 100% safe.
We therefore advise that you give access to Flow creators with this in mind.
We plan to change the architecture for script code evaluation in the future. If this is important to you now, please contact us to discuss.